- Published on
Code of the Lion
- Authors
- Name
- Jason Deramo
► A simple game that teaches beginner Python skills for all levels.
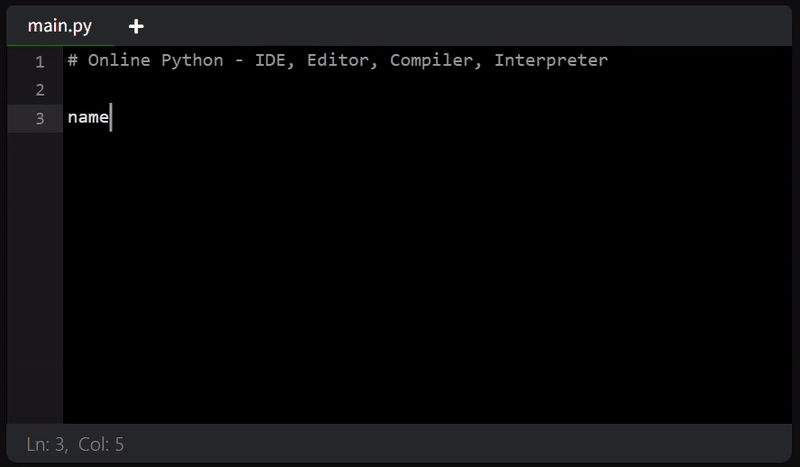
Hello World! Introduction
My name is Leo, your friendly lion cub. I need your help because I'm a bit lost in the jungle. I'm supposed to be the King, or at least in training. There are many surprises and dangers lurking around these parts. Will you help me find my way back home? Oh, and keep this between us for now ("Lion's Code").
\|\||
-' ||||/
/7 |||||/
/ |||||||/`-.____________
\-' ||||||||| `-._
-||||||||||| |` -`.
|||||| \ | `\\
|||||\ \______...---\_ \ \\
| \ \ | \ | ``-.__--.
| |\ \ / / | | ``---'
_/ /_/ / __/ / _| |
(,__/(,__/ (,__/ (,__/
Launch a Code Editor
Select the deploy button to open your terminal environment. Download "Code of the Lion" eBook for more detailed instructions and visual guides.
Lab 1: Welcome to the Jungle!
Learn: Print statements, Variables
Goal: Print a welcome message with Leo's name and age (e.g., "Hello! I'm Leo, a 2-year-old lion cub").
leo_name = "Leo"
leo_age = 2
print(f"Hello! I'm {leo_name}, a {leo_age}-year-old lion cub.")
Lab 2: River Crossing
Learn: Input function, Conditional statements (if/else)
Goal: Help Leo decide how to cross the river.
has_bridge = input("Is there a bridge? (True/False): ").strip().lower() == 'true'
if has_bridge:
print("Leo crosses the river safely.")
else:
print("No bridge! Help Leo find a log.")
Lab 3: Log Rolling
Learn: Loops (for)
Goal: Loop through 5 logs and check if one is suitable.
logs = [2, 4, 1, 3, 5] # Length of the logs in meters
suitable_log = None
for log in logs:
if log > 3:
suitable_log = log
print(f"Leo can use the {log}-meter long log.")
break
if suitable_log is None:
print("No suitable log found!")
Lab 4: Monkey Mayhem!
Learn: Functions
Goal: Create a function to reduce the banana count.
bananas = 5
def monkey_steal(banana_count):
return banana_count - 2
bananas = monkey_steal(bananas)
print(f"Leo now has {bananas} bananas.")
Lab 5: Helpful Toucan
Learn: Lists, String manipulation
Goal: Print each direction with an arrow.
directions = ["North", "East", "South", "West"]
for direction in directions:
print(f"{direction} ->")
Lab 6: Memory Test
Learn: While loops
Goal: Help Leo remember the toucan's directions.
correct_direction = "North"
user_direction = ""
while user_direction != correct_direction:
user_direction = input("Which direction did the toucan say? ").strip()
if user_direction == correct_direction:
print("Correct!")
else:
print("Try again.")
Lab 7: Fruit Feast
Learn: Random numbers
Goal: Random fruit from a tree.
import random
fruit = random.randint(1, 3)
if fruit == 1:
print("Leo found a delicious mango!")
elif fruit == 2:
print("Yum! Leo found a banana.")
else:
print("Leo enjoys a handful of sweet berries.")
Lab 8: River Rescue
Learn: String formatting (f-strings), lists, conditionals (if/else)
Goal: Help Leo rescue baby monkeys.
monkey_names = ["Lulu", "Milo"]
print("Oh no! Monkeys, Lulu and Milo, are stranded on opposite sides of the river!")
# Let the user choose who to rescue first
while True:
choice = input("Who will Leo save first? (1. Lulu or 2. Milo) ").upper()
if choice in ("1", "LUULU"):
rescued_monkey = "Lulu"
break
elif choice in ("2", "MILO"):
rescued_monkey = "Milo"
break
else:
print("Invalid choice. Please choose 1 or 2.")
# Print rescue message with f-string
print(f"Leo bravely swims across the river and rescues {rescued_monkey}!")
Lab 9: Cave Challenge
Learn: Dictionaries
Goal: Create a dictionary for cave exits.
cave_exits = {
"North": "dark and scary",
"East": "bright and sunny",
"South": "full of bats (yikes!)",
"West": "a narrow passage leading deeper into the cave"
}
user_choice = input("Leo stands at a crossroads in the cave. Which direction will he go? (North, East, South, West) ").capitalize()
if user_choice in cave_exits:
print(f"The exit to the {user_choice} looks {cave_exits[user_choice]}.")
else:
print("That direction seems like a dead end. Better choose another path!")
Lab 10 (Bonus): The Wise Old Elephant
Learn: Data Structures (Lists, Tuples)
Goal: Solve riddles with Lists and Tuples.
# A list of riddles and their corresponding answers as tuples
riddles_and_answers = [
("I have cities, but no houses. I have mountains, but no trees. I have water, but no fish. What am I?", "Map"),
("I am always coming, but never arrive. I am always present, but never here. What am I?", "Tomorrow")
]
user_score = 0 # Track Leo's score
# Loop through riddles and present them to the user
for riddle, answer in riddles_and_answers:
print(f"\nThe wise old elephant asks:")
print(riddle)
guess = input("What is your guess? ")
if guess.lower() == answer.lower():
user_score += 1
print(f"You're right! That's {user_score} out of {len(riddles_and_answers)} riddles solved.")
else:
print(f"Not quite. The answer is {answer}.")
# Share the overall lesson learned
learned_lesson = "We all need help in our journey... 'Keep going, you're doing great!'"
print(f"\nLeo ponders the lesson: {learned_lesson}.")